Qt Signal Slot Passing Array
What I want to know is how can I pass string based parameters to that same slot so that the slot knows which is this signal coming from. One alternative is to make as many slots as there are signals and then connect them in a 1:1 manner, but this is efficient, considering that the code for all the processing is very similar. Signals & Slots Signals and slots are used for communication between objects. The signals and slots mechanism is a central feature of Qt and probably the part that differs most from the features provided by other frameworks. Signals and slots are made possible by Qt's meta-object system. Try using Qt data structures instead of a simple array. Something like QList or QVector. They are already a metatype and can be sent by default through signals and slots. Let me know if it works. Qt::AutoConnection: If the receiver lives in the thread that emits the signal, Qt::DirectConnection is used. Otherwise, Qt::QueuedConnection is used. The connection type is determined when the signal is emitted. Qt::DirectConnection: This slot is invoked immediately when the signal is emitted. The slot is executed in the signaling thread.
- Qt Signal Slot Passing Arrays
- Qt Signal Slot Passing Array Calculator
- Qt Signal Slot Passing Array C++
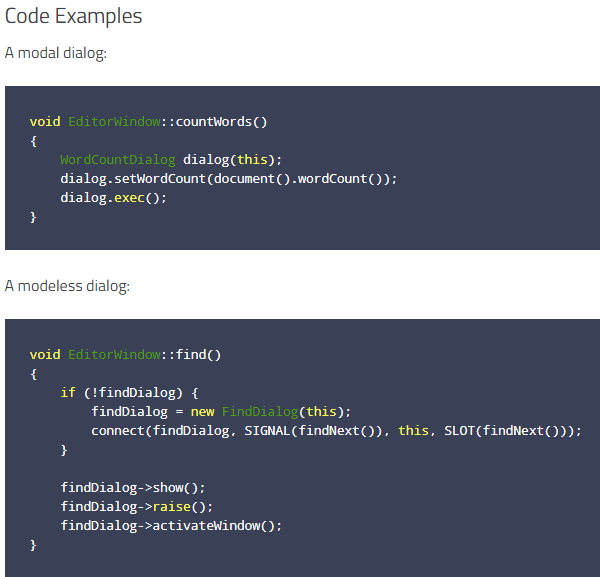
My GUI consists of a LineEdit and a PushButton. When the PushButton is clicked, the slot
I see two solutions:
Grant doSomething() access to the UI.
I want to be able to pass a QString to doSomething() as an argument. clicked() does not accept any arguments. Qsignalmapper might be what I'm looking for. However, it seems like the mapper only passes arguments based on what the signal is. The arguments therefore need to be setup in advance and it seems like it does not help me here.
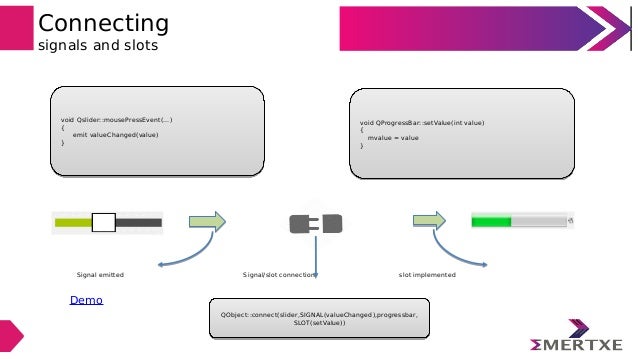
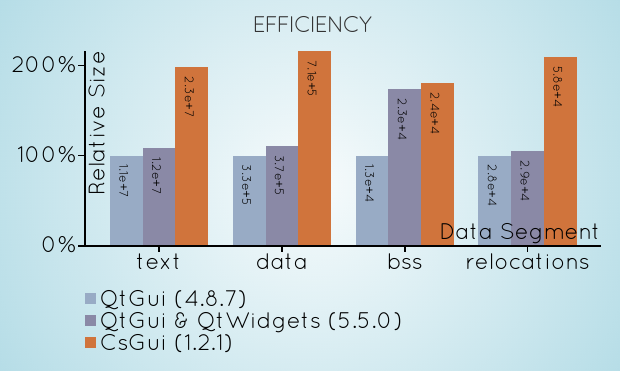
How would I do solution 2?
Assuming a C++ solution, you might consider using an intermediate signal like the following for #2:
connect(thisWidget, SIGNAL(clicked()), thisWidget, SLOT(slotClickHandler())); |
then:
void slotClickHandler() { QString s ='my variable string here'; emit sigDoSomething(s); } |
and them:
Qt Signal Slot Passing Arrays
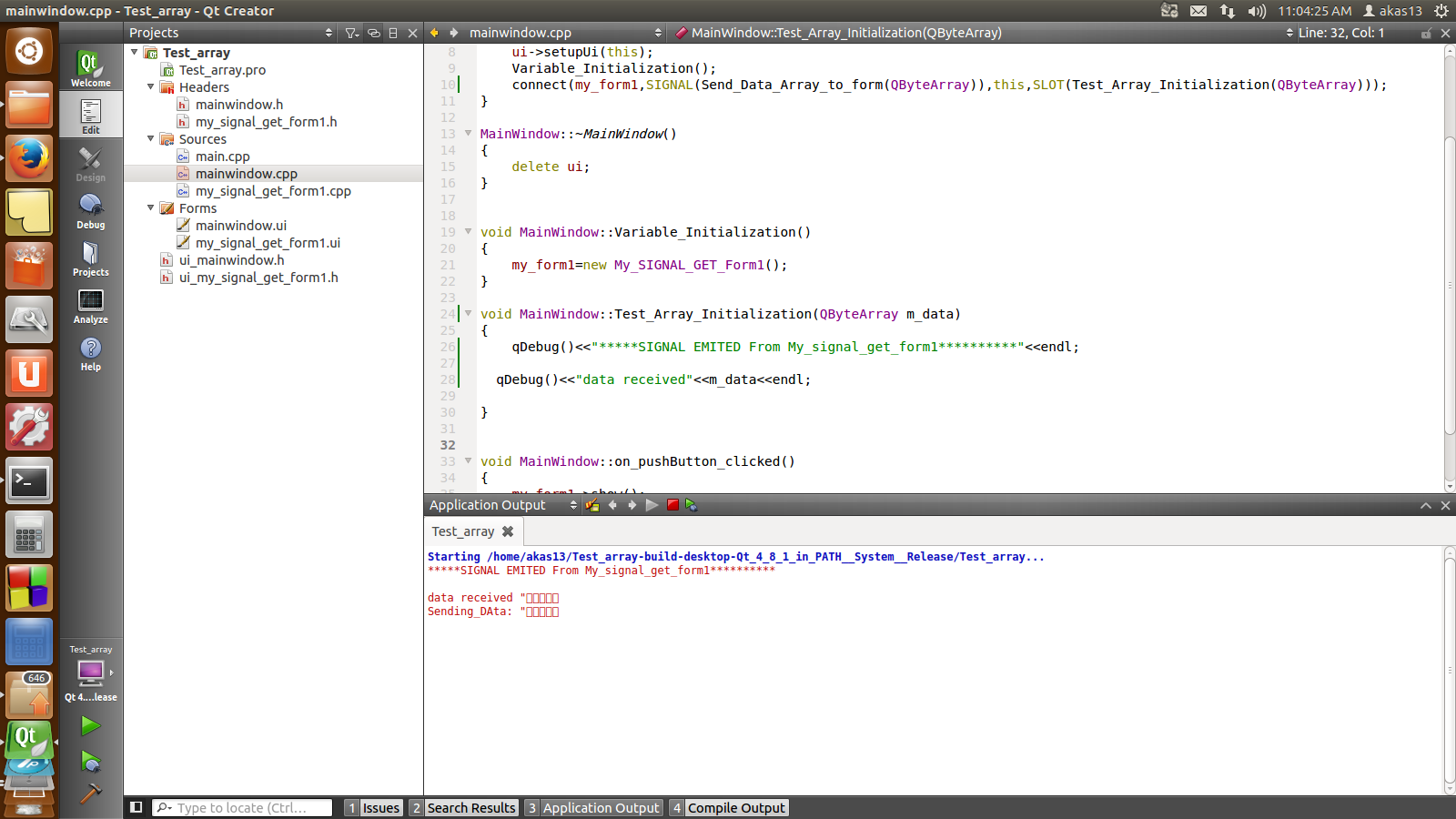

void slotDoSomething(const QString &s) { // Do something interesting with s here } |
Qt Signal Slot Passing Array Calculator
Note that this snippet assumes you've defined a new signal
Qt Signal Slot Passing Array C++
- Brilliant! Which would you say is cleaner? Method 1 or method 2? It depends, right? The problem with method 2 is duplicating the number of signals/slots can get messy when you're dealing with a lot of GUI elements, correct? Also, why is it const QString &s? Shouldn't it just be QString s?
- As you state, which is 'cleaner' depends. What does cleaner mean? Easier to read? Easier to debug? Easier on the compiler? The developer is usually the weakest link, so bias 'cleaner' accordingly. Map signals and slots clearly to their respective widgets (with meaningful names, etc.). Let the QT moc and gcc worry about the number of calls. Simple chained function calls will likely be optimized anyway. Lastly, the seeming duplication of calls will be dependent on your implementation: how many pushbuttons are there? what exactly do they need to convey>?, etc.
- As for const QString &, I'm assuming that the slot implementation does not need to modify the QString s. If so, a pass-by-value (i.e. QString s), will make a needless copy of the QString object. On the other hand, passing the QString by reference (QString &) allows the compiler to pass a more efficient reference (in essence a pointer to the object). Adding the const qualifier assures that the compiler is aware that the QString is not expected to be modified. (Indeed, the compiler will assert an error if you attempt to modify a const object).
- If you wish the slot to modify the QString, then you probably need to rethink the design. Nasty issues can arise.